Sending and receiving ICQ messages using PHP. Usage and application of PHP class WebIcqLite
In this articles we'll cover sending and receiving messages to/from ICQ users. This is a very useful way of communication with various applications. Lots of people have their ICQ client on for the whole day. Sending them an ICQ message is faster than an email - just it doesn't require login and so on every time you have to read a message (which is the problem ot the most free email services). So ICQ gives a fast and convenient way of reading newsletters and other applications. Of course this must not lead to abuse and sending thousands of spam messages.PHP class WebIcqLite
Since PHP has no built-in functions for interecting with ICQ network we'll user the class WebIcqLite. It can be obtained from http://intrigue.ru/workshop/php2icq.html (sorce code can be found on http://intrigue.ru/projects/icq/WebIcqLite.class.phps). It uses a real ICQ account, so get get a new one. The address is https://www.icq.com/register/ and registration is free.Here's a list of this class' most important members:
$error - variable holding the error of the last operation
connect($uin, $pass)
Tries to login the specified account. On success returns true, on failure - false and the error is recorded in $error.
get_message()
Waits for receiving a message. If succeeds, it returns an array with two elements. The one indexed with 'from' is the ICQ number of the sender and the other one (indexed with 'message') is the text of the message.
Example:
$mess=$icq->get_message();
echo "Received ".$mess['message']." from ".$mess['from'];
send_message($uin, $message)
Sends the message $message to the user $uin. On success returns true, on failure - false and the error is stored in $error.
disconnect()
Logout the account
Important characteristics
If you would like to send message in cyrillic, then you should encode it to windows-1251 charset.Example 1: Newsletter
An original way of sending newsletter (of course a small one with no html elements) is using ICQ, not regular email. There are certain things to have have in mind. ICQ server limits the connection attempts and sent messages for a time span. This means that you shouldn't start your script too often. Also your script shouldn't send messages too often. Here's the code itself:<?php
require "WebIcqLite.class.php";
$username='1234567890'; // put here the login details of your account
$password='password';
$newsletter_subscribers=array(
'11111111', '22222222', '333333333' // list with recipients
);
$newsletter_text='Check out the new ICQ article at http://ustrem.org/'; // message text
$icq = new WebIcqLite();
if(!$icq->connect($username, $password))
{
die($icq->error);
}
foreach ($newsletter_subscribers as $subscriber)
{
$result=$icq->send_message($subscriber, $newsletter_text);
if (!$result)
{
echo "\nCannot send to $subscriber, reason: ".$icq->error;
}
else
{
echo "\nSent to $subscriber";
}
sleep(30);
}
$icq->disconnect();
?>
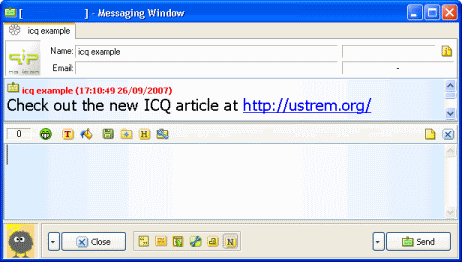
Example 2: Lost password
Recovering of password is a long and boring activity both for the user and the administrator. Standard procedure is sending it to email, or sending a verification code and so on. But if we assume the user has registered his/her ICQ number (and if you rely on ICQ security), this whole process can be replaced by a simple message:<?php
ignore_user_abort(true);
set_time_limit(0);
require "WebIcqLite.class.php";
$username='1234567890'; // put here the login details of your account
$password='password';
$icq = new WebIcqLite();
if(!$icq->connect($username, $password))
{
die($icq->error);
}
// change this function so that it extracts the password of you user based on his/her ICQ number
function get_password_for_icq_number($str)
{
return $str;
}
while ($message=$icq->read_message())
{
$password=get_password_for_icq_number($message['from']);
if ($password)
{
$icq->write_message($message['from'], 'Your password is '.$password);
}
else
{
$icq->write_message($message['from'], 'This ICQ number is not registered');
}
}
?>
Some explanations are needed here. Since this script should be running all the time, it cannot be used via a webserver. The best practice is to run it directly from a PC with PHP installed. This can be done either on Linux or Windows box with a simple command:
>php file_name.php
Both functions ignore_user_abort() and set_time_limit() ensure that the script won't be terminated due to timeout.
Example 3: Auto responder
Here's a bit different application. This is an auto responder script, that sends back different messages for different users. It can be complicated so that it becomes something similar to an IRC bot. However let's keep it simple. Tis script should be run similar to the previous one:<?php
ignore_user_abort(true);
set_time_limit(0);
require "WebIcqLite.class.php";
$username='1234567890'; // put here the login details of your account
$password='password';
$message1='I am not at home';
$message2='I am at John\'s. Meet you there';
$message2_recipients=array('11111111', '22222222');
$icq = new WebIcqLite();
if(!$icq->connect($username, $password))
{
die($icq->error);
}
while ($message=$icq->read_message())
{
if (in_array($message['from'], $message2_recipients))
{
$icq->write_message($message['from'], $message2);
}
else
{
$icq->write_message($message['from'], $message1);
}
}
?>
These were a few limited examples to show you haw to work wit WebIcqLite. You can however extend them or write completely new ones to satisfy your needs.
Comments:
medhi (08-10-2007 02:14) :
Hi, what about waiting for read_message? PHP script is waiting, until some message is received. But this is not so good, I think.
Cant be better, if read_message was just look for some new messages and then close?asminog (13-02-2008 16:45) :
http://intrigue.ru/workshop/webicqpro/webicqpro.html
- more functions then Lite.
Simple bot included.sever (20-06-2008 14:38) :
i'm using this to communicate through icq and several other protocols
http://thinker.rubay.de/archives/20-PHPurple-v0.2-alpha-released.htmlPaul (30-10-2008 00:45) :
Is there a tutorial for using the webicqpro or any other class that I could use for getting the friends of a icq user?Adel (18-02-2009 07:04) :
Is there any way to stay online forever?!
because icq will ban userid after 2-3 connectionro0ne (27-03-2009 18:52) :
Example 3 stays online for ever.Stobe02 (15-05-2009 18:07) :
Best class I've seen for long time.ROI (26-08-2009 16:31) :
there is a time out ?
and if there is, how can i remove it ?Eugene (05-11-2009 12:46) :
What happens if by some reasson connection breakes? How can I make my script track the connection status and reconnect if needed?Alex (06-06-2011 01:08) :
Take a look at my service based on this class - sendicq.inbelarus.net
you may send icq-message directly from webalex (06-10-2011 20:04) :
Working online icq service that allows to send messages - http://sendicq.inbelarus.net/
It's based on described webicqlite class.
This page was last modified on 2025-07-10 23:57:42